之前一直是通过matplotlib来画图的,数据量大了以后,画图的速度较慢。
如果使用plotly,效率较高,主要是通过浏览器来渲染图片的,通过js效果,还可以随意放大缩小查看细节。
基本绘图
折线图
1 | import plotly.express as px |
或者更简便一些
1 | import plotly.express as px |
柱状图
1 | px.bar(df["pnl"]) |
散点图
1 | px.scatter(df["drawdown"]) |
对象方式绘图
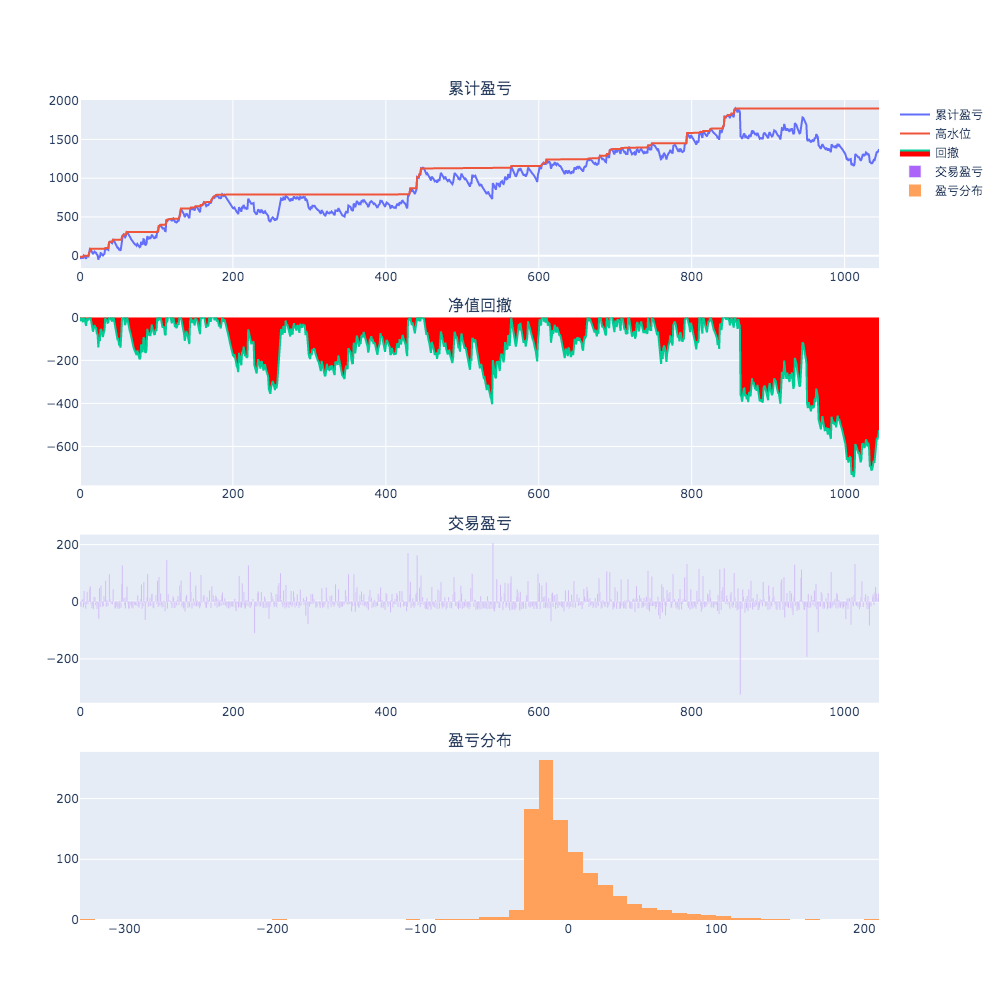
创建绘图区域
1 | import plotly.graph_objects as go |
创建四幅子图
Scatter取代了Line
1 | # 绘制资金曲线 |
这个有填充的效果
1 | # 绘制回撤区域 |
1 | # 绘制交易盈亏 |
1 | # 绘制盈亏分布 |
把子图添加到画布上面
1 | # 绘制图表 |